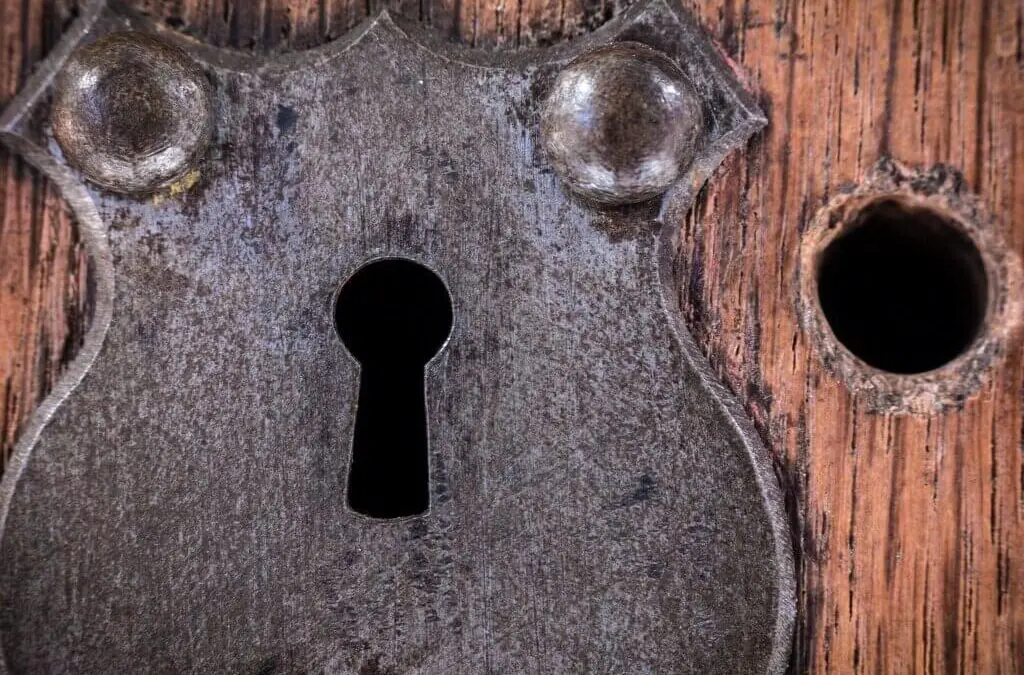
Google API Authorization with Ruby using long lasting Refresh Token
Authorization is the most important thing when it comes to API integration.
There are multiple ways for authorization, based on whom your code access Google on behalf of:
- On Behalf of yourself (Command line authorization).
- On Behalf of the user who accesses your web app (Web-based authorization).
- On Behalf of no existing users (Service account).
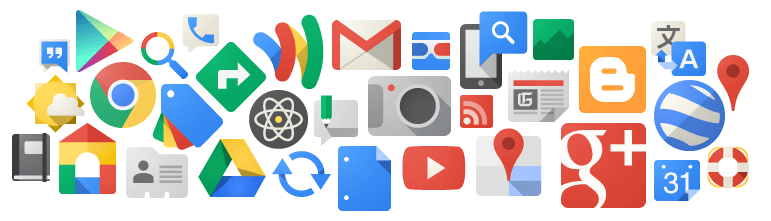
Today we will see how to achieve Web-based authorization. There are two ways with the help of which we can implement this.
How to do it?
With auth_url
- When the application needs to access a user’s data, redirect the user to Google’s OAuth 2.0 server, Google’s OAuth 2.0 server authenticates the user and obtains consent from the user for your application to access the requested scopes. The response is sent back to your application using the redirect URL you specified.
- But here user intervention is frequently required to grant your application the requested access
With Long-lasting refresh token
- Access token expires in every 60 min, so you need to refresh it every time. We can get long-lasting refresh token and use it to generate new access token as and when we need.
- unlike auth_url option, frequent user intervention is also not required here
Today We will be looking at, how authorization can be implemented with long lasting refresh token. So here we Go! We will use the Google API client Ruby to see how long lasting refresh token can be used.
Step-1: Create a Web app and obtain refresh token and other credentials.
This can be done with the Oauth2 Playground at https://developers.google.com/oauthplayground.
Then,
- Create the Google Account (eg. my.drive.app@gmail.com)
- Use the API console to register the mydriveapp (https://console.developers.google.com/apis/credentials/oauthclient?project=mydriveapp or just https://console.developers.google.com/apis/)
- Create a new set of credentials (NB OAuth Client ID not Service Account Key and then choose “Web Application” from the selection)
- Include https://developers.google.com/oauthplayground as a valid redirect URI
- Note the client ID (web app) and Client Secret
- Login as my.drive.app@gmail.com
- Go to Oauth2 playground
- In Settings (gear icon), set
- Oauth flow: server
- Access type: offline
- Use your own OAuth credentials: TICK
- Client Id and Client Secret: from step 5
- Click Step 1 and choose Drive API https://www.googleapis.com/auth/drive (having said that, this technique also works for any of the Google APIs listed).
- Click Authorize APIs. You will be prompted to choose your Google account and confirm access
- Click Step 2 and “Exchange Authorization code for tokens”.
- Copy the returned Refresh Token and paste it into your app, source code or into some form of storage from where your app can retrieve it.
Now we have our credentials Client Id, Client Secret and refresh token with us from the Google API client library for Ruby.
Lets write some ruby code to get it to work…
Here we will be using the googleauth library, so create a simple Google API client ruby file and include this library.
require "googleauth"
Now we have to create an instance of Google::Auth::UserRefreshCredentials
so set credentials for our user,
and done! Now you can use this credential instance to authorize any of the Google service you wish to use.
You can use this as follows, here I am using Google Drive API so we need to add this first.
That’s it guys, and now we can use a service object to call any of the services of Google Drive API.
So our final code will look like:
That’s it! Happy coding!!!
Consulting is free – let us help you grow!