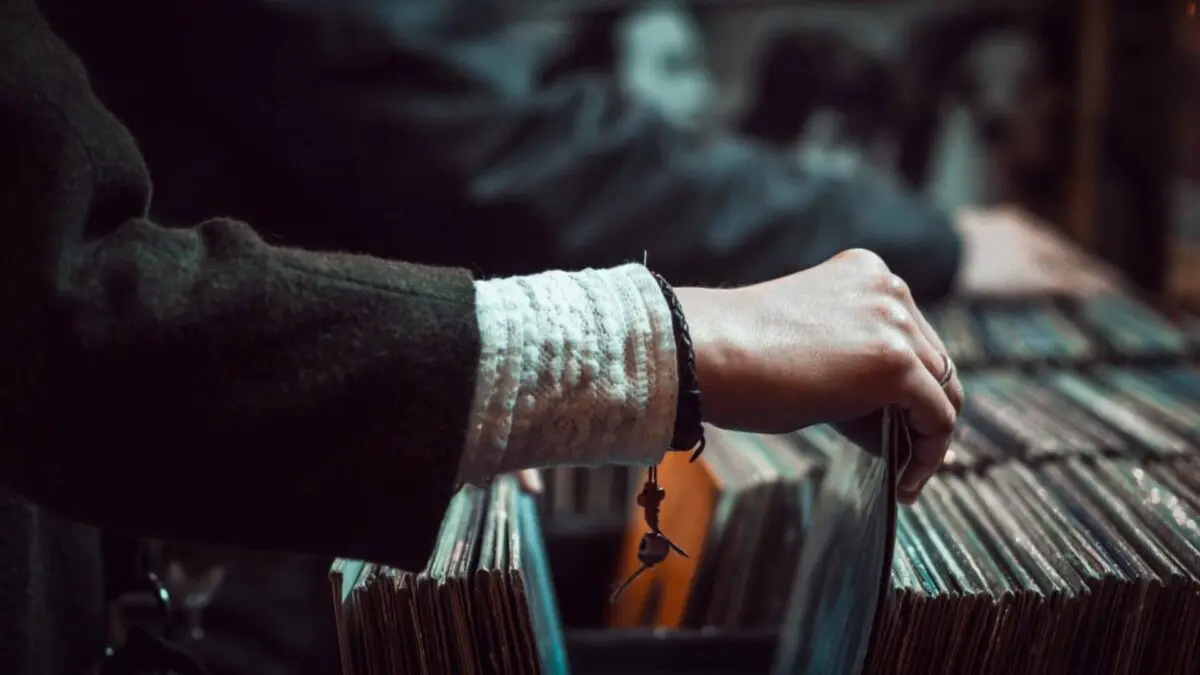
Sort Rails Records Using Enum Value
Ransack empowers rails developers by enabling the creation of simple and advanced search forms in any Ruby on Rails application.
If we are dealing with simple sort by column then it is very easy to use however if we have Enum but not in the order in which we want to sort is painful.
Let’s take an example,
class ClientVisit < ApplicationRecord
enum status: [:inprocess, :completed, :incomplete, :resubmit]
end
Here we have a status enum
where the index of each status defined as,
{ inprocess: 0, completed: 1, incomplete: 2, resubmit: 3 }
Now if you try to sort status column using helper method sort_link, it will always sort the data in its enum
value order (i.e., 0, 1, 2)
STATUSES = [:inprocess, :completed, :incomplete]
ClientVisit.where(status: STATUSES).ransack(params[:q]).result
QUERY OUTPUT
SELECT "client_visits".* FROM "client_visits"
WHERE "client_visits"."status" IN (0, 1, 3) "client_visits"."status" ASC
SO
FOR "ASC" the order will be => [ 0, 1, 2 ]
FOR "DESC" the Order will be => [ 2, 1, 0 ]
Now, if you want to order by status with specific order like sort by status in order:
1. Completed.
2. InComplete.
0. InProcess.
Now, please note here, we CAN NOT override the default sort. Instead, we can create custom scope and column which will fulfill our requirement.
First, go to view file and use a custom column name:
Ex.
Previous:
<%= sort_link(@q, :status, "STATUS", { action: 'client_visit' }, { remote: true }) %>
Updated
<%= sort_link(@q, :custom_status, "STATUS", { action: 'client_visit' }, { remote: true }) %>
Here is a gist of the model with the implementation:
Now in the controller,
Query Output would look like this,
ASC
SELECT "client_visits".* FROM "client_visits"
WHERE "client_visits"."status" IN (0, 1, 2)
ORDER BY CASE WHEN status=1 THEN 0 WHEN status=2 THEN 1 WHEN status=0 THEN 2 END, "client_visits"."status" ASC
DESC
SELECT "client_visits".* FROM "client_visits"
WHERE "client_visits"."status" IN (0, 1, 2)
ORDER BY CASE WHEN status=2 THEN 0 WHEN status=2 THEN 1 WHEN status=1 THEN 2 END, "client_visits"."status" DESC
That’s what we are expecting. Congratulations!!!
At BoTree Technologies, we build enterprise applications with our RoR team of 25+ engineers.
We also specialize in RPA, AI, Python, Django, JavaScript and ReactJS.