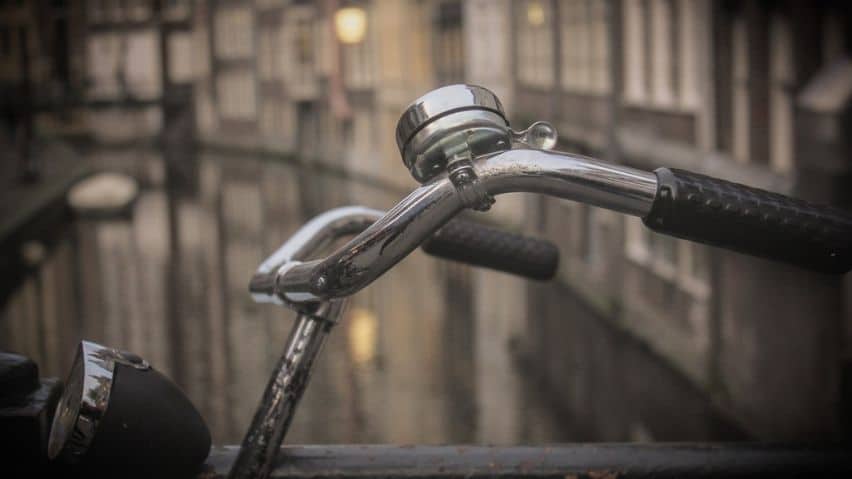
Using Handlebars JS with Ruby on Rails!
What is Handlebars.js?
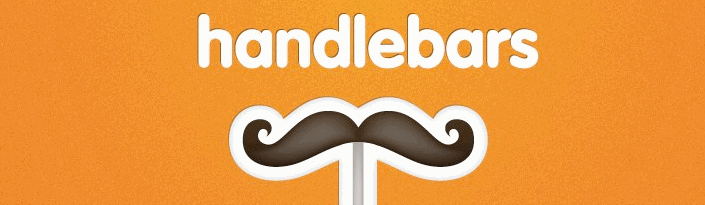
Handlebars allows to build semantic templates. Handlebars is largely compatible with Mustache templates. In most cases it is possible to swap out Mustache with Handlebars and continue using your current templates.
Official Handlebars Docs: http://www.handlebarsjs.com
Live demo: http://tryhandlebarsjs.com/
Now let’s get started!
Prerequisites
Before you get started, make sure you have RVM, Ruby and Rails installed on your machine. If you don’t, please follow the instructions here: How To Install Ruby on Rails on Ubuntu.
Below version of Ruby and Rails were used in this tutorial
Rails 5.0.2
Ruby 2.2.3
You can find the full source code of this tutorial handlebar-rails.
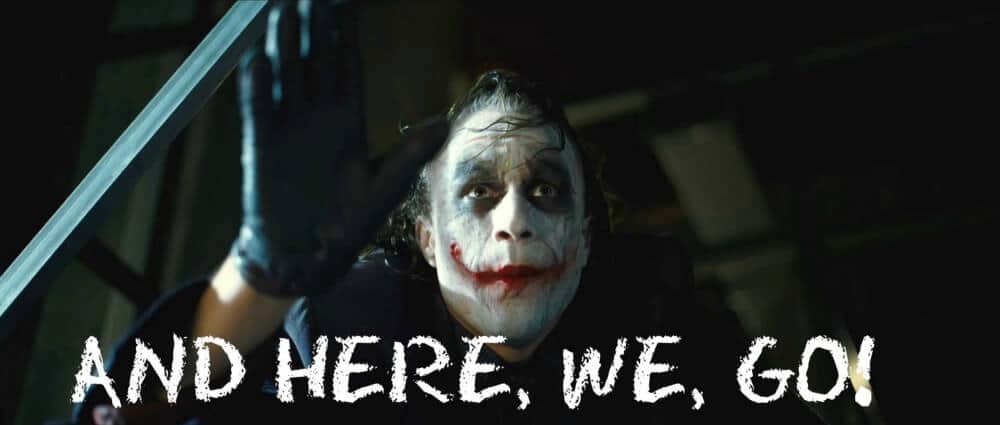
Start by creating brand new rails app.
$ rails new handlebar-rails
Add bootstrap gem to apply some styling to your pages. Of course this is optional!
Let’s create the API for the books model which we will use in this tutorial.
$ rails g scaffold Book name price:integer author - skip-template-engine
Adding –skip-template-engine will create API without html views.
As we would use books routes as an API only, we set default format as JSON in config/routes.rb file of our app. Doing so will send all the request as JSON.
resources :books, defaults: {format: :json}
Now let’s add handlebars gem to our Gemfile.
gem 'handlebars_assets'
and then do $ bundle install
so it gets installed in the rails app.
As we are working with semantic JS templates they need to be organized in assets/javascripts folder.
Create directory named templates in the assets/javascripts folder as suggested in the official documentation of the handlebars gem. So it will have the below structure.
assets/javascripts/templates
Now add following to your application.js manifest file.
//= require handlebars
//= require_tree ./templates
Note: Make sure you keep it before //= require_tree .
else you will be running into major problems.
Now let’s add our first Handlebars template book_list.hbs to our app.
One new thing that you will notice here is {{}} block, anything written in this block is considered as dynamic code and will be evaluated by handlebars at run-time. It is similar to the code written as “#{a+b}” which is termed as string interpolation in ruby.
Here all the code written between {{#books}} … {{/books}} is looped on the books object. Handlebars provide some built-in helpers so we will use them right out of the box.
{{#books}}
<td>{{name}}</td>
<td>{{price}}</td>
<td>{{author}}</td>
{{/books}}
Above will treat name as book.name as it is looped within books object loop. Same applies to price and author.
Any handlebar templates can be invoked using
HandlebarsTemplates[<template_name>](context)
As for example,
HandlebarsTemplates['book_list']({ books: data })
Here it searches assets/javascripts/templates/book_list.hbs template and pass contents of data as books object to the template.
You can also check html output of the same in console of your web browser. That’s pretty cool!
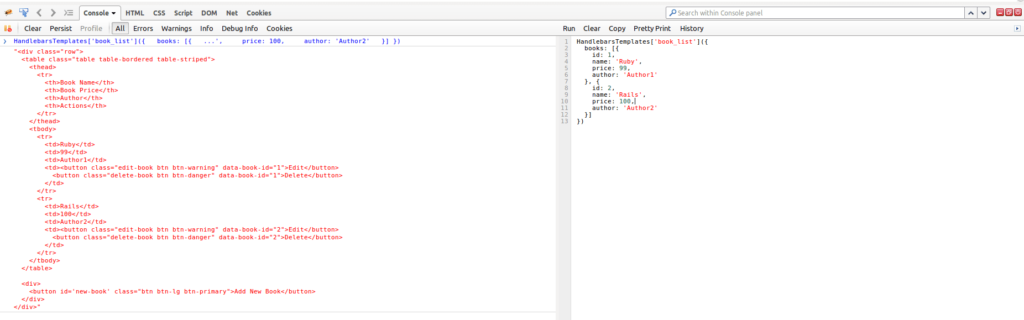
In our rails app, on the home page load below AJAX call gets fired.
This call gets all the data of books in JSON format and pass it as context to book_list handlebars template. The html will be generated based on the data passed is stored in bookListHtml variable. Then it finds the div with the id named book-list and replace the html with newly generated handlebar html template.
So handlebars makes it easy to generate views with JavaScript only. Add, edit and delete functionality has been also implemented using handlebars templates, you can find the source code at handlebar-rails. See below to have a look at what is implemented!

Handlebars also support haml and slim templates do explore it!
That’s it! Thanks for reading. If you liked it, press the lovely heart button 🙂
At BoTree Technologies, we build enterprise applications with our RoR team of 25+ engineers.
We also specialize in RPA, AI, Python, Django, JavaScript and ReactJS.
Consulting is free – let us help you grow!
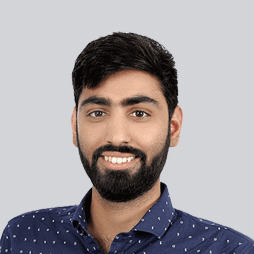