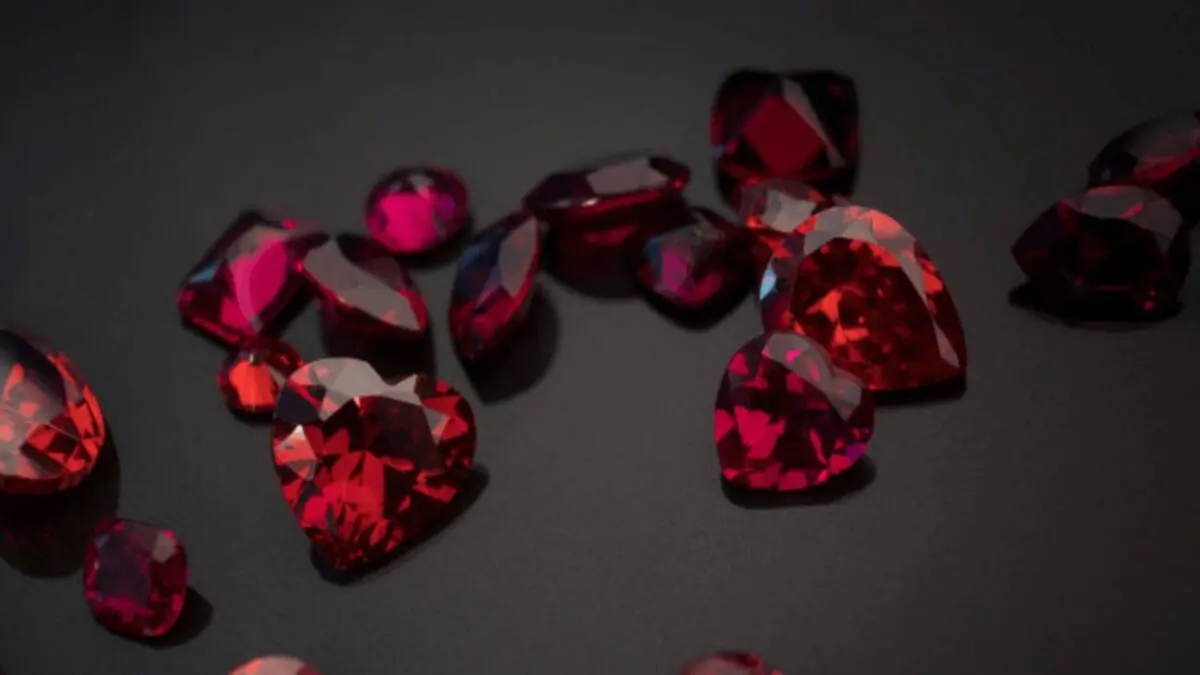
Ruby Best Practices to Follow in Daily Coding
A cleanly written code is more easy to understand
As beginner I always used to do common mistakes while writing the code. There are few best practices which one must follow while writing the Ruby code.
Both technical and non-technical best practices are important to ensure that the code runs smoothly. Ruby is a dynamic programming language that requires attention. While most mistakes can be let go, not following Ruby best practices will lead to future problems. You might get stuck in the coding process or forget entirely about a feature because it required a simple algorithmic approach.
To avoid such mistakes, Ruby encourages developers to follow some best practices that ensure the product is flawless, to some extent. Let’s have a look at some technical and general best practices of Ruby in detail.
Checkout Top 5 best Ruby on Rails projects developed by BoTree Technologies
General Ruby best practices
Most developers get so engrossed in technicalities and coding that they forget about the general behaviour of Ruby and its frameworks like Ruby on Rails. Here are a few general best practices for Ruby –
- Follow community guidelines
If you want to avoid the basic mistakes in Ruby, it is important to follow the standard community guidelines. It will help you to build a consistent coding style and base. When there are different people working on a project, everyone will be able to align with each other in a better way. - Don’t Repeat Yourself
It is widely known that avoiding duplication in Ruby is one of the best ways to efficiently build products. Reuse components and codes wherever possible – it reduces the development time drastically. Follow the general Ruby principles to avoid using the code in Ruby on Rails and other frameworks. - Convention over configuration
If you follow the principle of convention over configuration, you will save yourself from a lot of trouble. Ruby is a powerful language that works well with Ruby on Rails. Therefore, follow the conventional development environment. The standard method will allow you to focus on coding rather than optimizing and configuring the framework. - Internationalization/localization
The best practice for Ruby is to integrate internationalization in the app from the beginning. If the app is targeting a diverse audience, then linguistic development is much better than others. It will save a lot of time and money in the future when you want to scale the application to reach audiences in different countries.
Technical Ruby best practices
Now that we understand the general best practices for Ruby, let’s move on to understanding the technical best practices for Ruby and its frameworks.
Always use spaces around operators, after commas, colons and semicolons, around { and before }. White space might be (mostly) irrelevant to the Ruby interpreter, but its proper use is the key to writing easily readable code. Also it makes the design more readable and code much cleaner.product = 1 * 2
array = [1, 2, 3, 4, 5]
array.map { |a| a + 2 }
There should be no spaces after (, [ or before ], ) these brackets.['ankur', 'vyas'] sum(a, b)
Also don’t use spaces in while providing the range.Use 5..9 over 5.. 9
Use 'a'..'z' over 'a'.. 'z'
When using switch case statements use the following indentation.
Use following indentation if the parameters exceeds to more than one line.
Use splat for better assignments.element_1, *other_elements =['a', 'n', 'k', 'u', 'r']
If only 2 conditions are present use the ternary operators.var_1 = condition ? assignment_1 : assignment_2
If you are setting a variable using the if condition the use following syntax.
Avoid return statements at the end of the line. In ruby the last line of the method is returned.
Use snake case for all the ruby variables.variable_1, some_variable
Use camel case for class names.ClassOne, ClassTwo
Use screaming snake case for the constants’ names.CONSTANT_1, CONSTANT_2
Use %w for the string arrays and %i for the symbol arrays.STATUS_MAP = %w(open closed draft paid)
SYMBOL_MAP = %i(symbol1 symbol2 symbol3)
Use string interpolation over concatenation.Use "#{string_1} < #{string2} >" over string_1 + '<' + string2 + '>'
Assign single quotes to the string which does not have interpolation.Use 'string 1' over "string 1"
Do not use the word partial when you don’t want to pass some variables in it.Use render 'partial_1' over render partial: 'partial_1'
So above were some simple tips which a Ruby Coder must follow.
Thank you for reading!
References:
bbatsov/ruby-style-guide
ruby-style-guide – A community-driven Ruby coding style guide
Originally published at vyasankur27.blogspot.in on July 24, 2016.
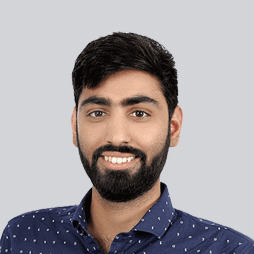