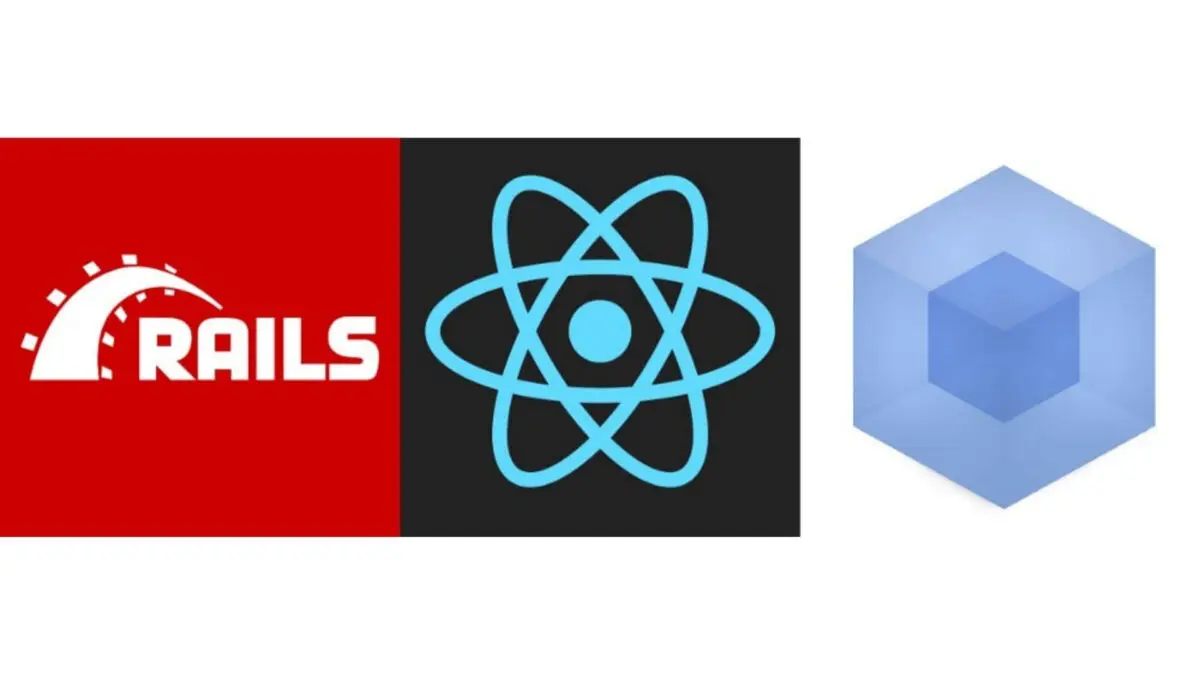
How to add React JS to your Ruby on Rails App with Webpacker
This tutorial will guide you on how to create a Ruby on Rails application and add the power of webpacker and React JS to it.
Let’s have a brief introduction to Webpacker and React JS. If you are familiar with React JS and Webpacker directly go to Implementation section.
What is React JS?
- React JS is a JavaScript library for building user interfaces and is developed by Facebook.
- React allows developers to create large web-applications that use data and can change over time without reloading the page.
- It aims primarily to provide speed, simplicity and scalability. React processes only user interfaces in applications.
- It corresponds to View in the Model-View-Controller (MVC) pattern.
You can find the list of sites that use React JS here. It includes Facebook, Instagram, Netflix, Khan Academy, Asana etc. to name a few.
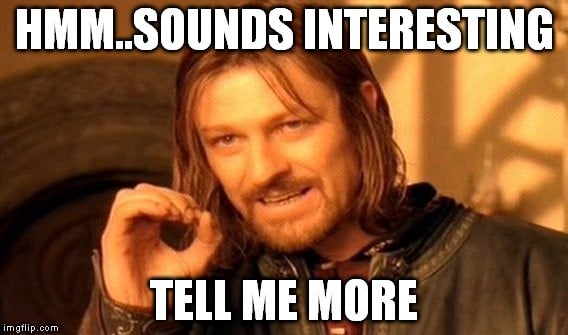
PC: https://imgflip.com/i/r8qh2
Why React JS?
- Simplicity: No complex two-way data flow.
- Uses simple one-way reactive data flow.
- Performance: React is fast!
- Real DOM is slow.
- JavaScript is fast.
- Using virtual DOM objects enables fast batch updates to real DOM, with great productivity gains over frequent cascading updates of DOM tree.
If your page uses a lot of fast updating data or real time data - React is the way to go.
That’s a small introduction about React JS.
What is webpacker?
At its core, webpacker is a static module bundler for modern JavaScript applications. It is responsible to bundle all our .jsx
, .sass
, .hbs
etc. code into normal js and css that our browser understands after necessary pre-compilation.
- Webpacker makes it easy to use the JavaScript pre-processor and bundler webpack 3.x.x+ to manage application-like JavaScript in Rails.
- It coexists with the asset pipeline, as the primary purpose for webpack is app-like JavaScript, not images, CSS, or even JavaScript Sprinkles (that all continues to live in app/assets).
- However, it is possible to use Webpacker for CSS, images and fonts assets as well, in which case you may not even need the asset pipeline.
- This is mostly relevant when exclusively using component-based JavaScript frameworks.
That was a small introduction to webpacker. Now, let’s start creating the rails app.
Technology Stack
- Technology: Ruby on Rails.
- Versions: Rails: 5.1.5 , Ruby: 2.4.1.
- Git Repo: https://github.com/AnkurVyas-BTC/rails-react-webpacker.
$ rails new rails-react-webpacker $ cd rails-react-webpacker
Add webpacker
and react-rails
to your Gemfile
and run the installers.
$ bundle install $ rails webpacker:install $ rails webpacker:install:react $ rails generate react:install
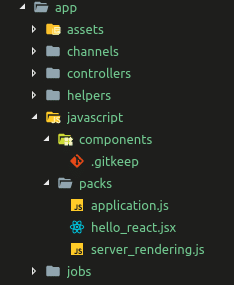
After the successful completion the of the above commands. You will have the directory structure like below image.
Javascript folder structure.
app/javascript/components/
directory.The content of app/javascript/packs/application.js
is as following.
The line var componentRequireContext = require.context("components", true)
makes the react components available to our rails app.
Now, we just need to add the above file into our application layout file at app/views/layouts/application.html.erb
.
Let’s create a home controller with index method. We will mount our first react component to app/views/home/index.html.erb
file.
$ rails g controller home index
Now, let’s create our first React Component.
rails g react:component GreetUser name:string
Running the above command will create our first react component at app/javascript/components/GreetUser.js
.
Now, replace contents of app/javascript/components/GreetUser.js
to match below.
In above file the GreetUser
component is expecting name
props.
Now, to add the component to view we need to use the react_component
helper. So change the contents of app/views/home/index.html.erb
to match below.
Now, this calls our GreetUser
component and passes the name
(here ‘Ankur
‘) as a props
to our component.
Now, visit localhost:3000/home/index
you should see the following screen.
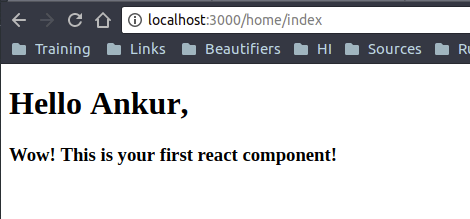
If you have added React Developer Tools to your browser. Then you must see the output as the following snap.
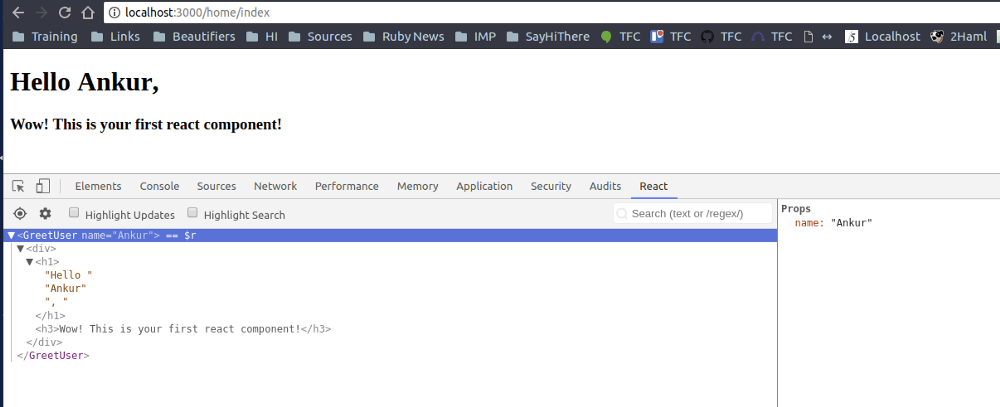
You can find the whole github code here.
So start using React JS with Rails and feel the difference!!!
At BoTree Technologies, we build enterprise applications with our RoR team of 25+ engineers.
We also specialize in RPA, AI, Python, Django, JavaScript and ReactJS.
Consulting is free – let us help you grow!
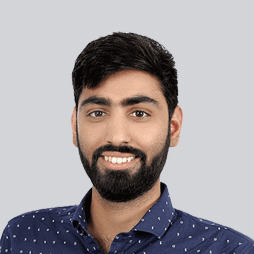