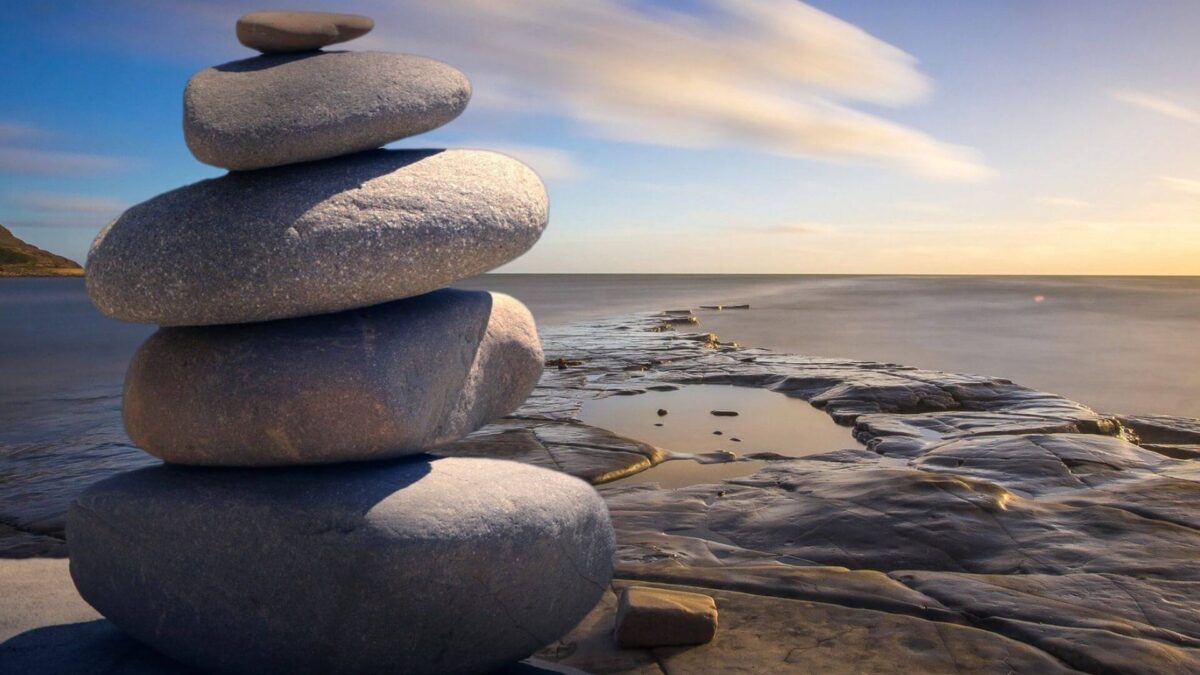
Integrate Redux Form in your React App – Part 1
What is Redux-form?
Redux Form works with React Redux to enable an html form in React to use Redux to store all of its state.
redux-form removes the headache to manage each input state individually in component, reduce total lines of code as well. so let’s start.
- React and HOC (Higher Order Components)
- Redux
Let’s start with create-react-app.
create-react-app react-redux-form
Install redux, redux-form packages.
npm install --save redux-form redux
… and start npm server.
npm start
create src/store.js as follow:
In above code you can see reduxFormReducer.
reduxFormReducer is function that tells how to update the Redux store based on changes coming from the application; those changes are described by Redux actions.
Now create a simple form using redux-form as follows. This is a simple demonstration of how to connect all the standard HTML form elements to redux-form.
create src/SimpleForm.js as follow:
In the above code, you can see we use <Field/> component from redux-form. The <Field/> component connects each input to the store. It creates an HTML <input/> element of type given type (text, select, etc…). It also passes additional props such as value, onChange, onBlur, etc. Those are used to track and maintain the input state under the hood.
Other one is reduxForm() which is a Higher Order Component (HOC), that takes configuration object and returns a new function; use it to wrap your form component and bind user interaction to dispatch of Redux actions.
So as a summary, We have a form component (SimpleForm.js) wrapped with reduxForm() There is one text input inside, wrapped with <Field/>. The data flows like this:
- User clicks on the input,
- “Focus action” is dispatched,
- formReducer updates the corresponding state slice,
- The state is then passed back to the input.
Same goes for any other interaction like filling the input, changing its state or submitting the form.
create src/showResults.js to view data when form is submitted.
Now edit src/App.js as follow:
That’s it. we have integrated redux-form in our react-app successfully…!
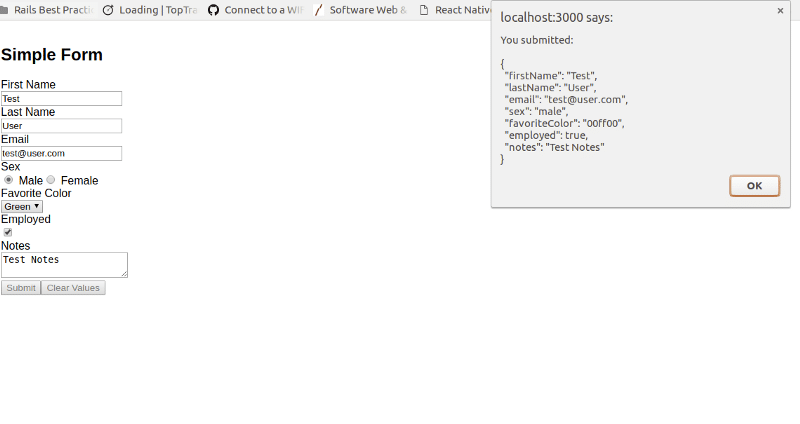
redux-form after submit
You can find the whole source code here.
With redux-form comes a lot more: hooks for validation and formatting handlers, various properties and action creators. we will learn about that in next part, till then enjoy coding…!
References.
At BoTree, we build web and mobile applications to add value to our client’s business. We align ourselves to ensure that our client benefits the most out of our engagement.
We work in Ruby on Rails, Python, Java, React, Android and iOS.
Drop us a line to discuss how can we help take your business to the next level.
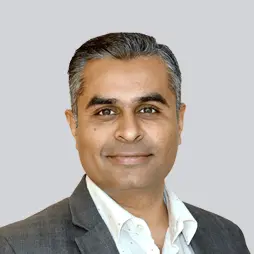