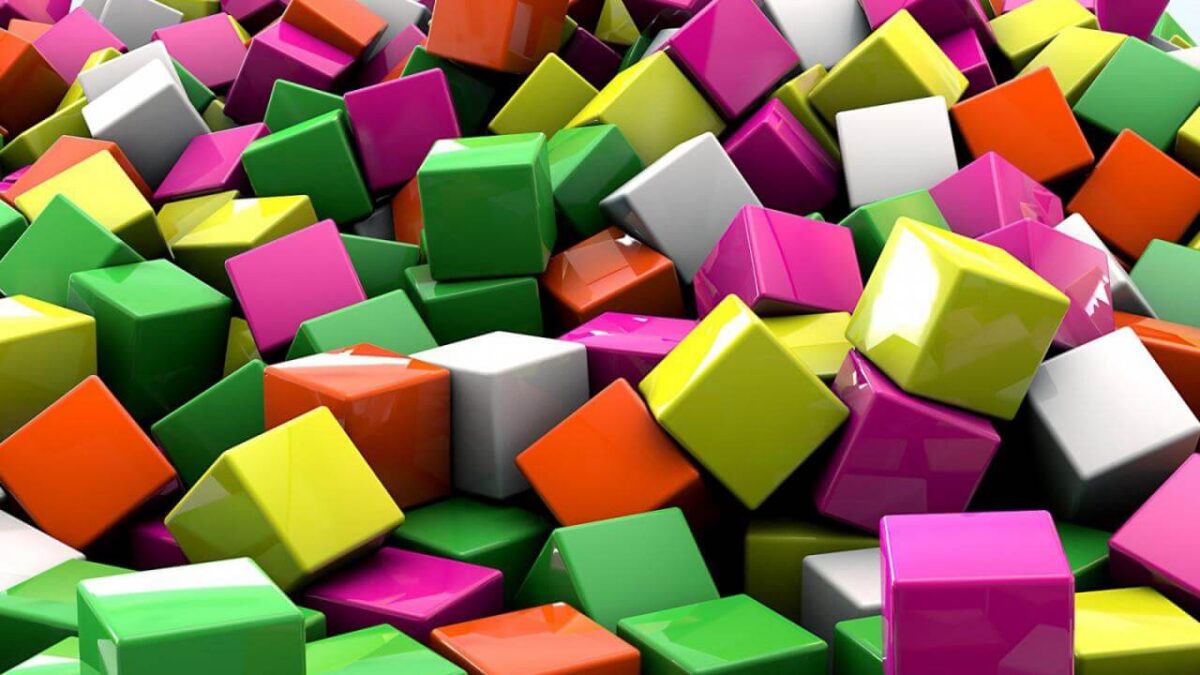
Understanding Basic Ruby Data Types
When we start learning a programming language, we generally start by learning about data types in ruby, variables, operators, conditionals, looping and then into more of oops principles. In this post, we will see what is ruby on rails data type and how we can define it by different ways.
Ruby has several data types. All ruby data types are based on classes. The following are the basic data types recognised in Ruby:
- Arrays
- Hashes
- Boolean
- Symbols
- Numbers
- Strings
Arrays
Ruby arrays are ordered collections of objects. They can hold objects like integer, number, hash, string, symbol or any other array.
An array is a list of variables enclosed in square brackets and separated by commas like [ 1, 2, 3 ].
Its indexing starts with 0. The negative index starts with -1 from the end of the array. For example, -1 indicates last element of the array and 0 indicates first element of the array.
There are many ways to create or initialise an array like…
Hashes
A Hash is a collection of key-value pairs like this: “employee” => “salary”. key-value pair has an identifier that identify which variable of the hash you want to access and a variable to store in that position in the hash.
Define Hash
A blank hash can be declared using two curly braces {}. Here is an example of a Hash in Ruby:
employee_ages = {
"John" => 31,
"David" => 25,
"Mike" => 23
}
The names (John, David, Mike) are the keys and 31, 25 ad 23 are the corresponding values.
Another way to define a hash when your keys are Symbols,
employee_ages = {:John => 31, :David => 25}
=> {:John=>31, :David=>25}
A Hash can also be created through its ‘new’ method:
employee_ages = Hash.new
employee_eges["John"] = 31
We can also set the default value that returns when accessing keys are not exists in the Hash. If not defined the default value then it returns nil. We can pass this as an argument to ::new.
employee_ages = Hash.new(0)
Fetch value from a Hash
You can retrieve values from a Hash using [] operator. Now, try finding the age of John from employee_ages hash, write the name of the object with square bracket and write key John inside the brackets. In this case the key is string so take the key in quotes.
employee_ages["John"]
=> 31
OR
employee_ages = {:John => 31, :David => 25}
employee_ages[:John]
=> 31
Booleans
In our code, we need the boolean values in regular basis. We are taking lots of decision based on true or false. true is an instance of TrueClass and false is an instance of FalseClass. Let’s see how the boolean used in Ruby.
Boolean Operators
In Ruby there are three main boolean operators:
- ! (“single-bang”) which represents “NOT”,
- && (“double-ampersand”) which represents “AND”, and
- || (“double-pipe”) which represents “OR”.
- == (“double-equal-sign”) which is for compare two values.
For an && (“and”) to evaluate to true, both values of either side of the symbol must evaluate to true. For example:
true && true #=> true
true && false #=> false
For an || (“or”) to evaluate to true, only one value on either side of the symbol must evaluate to true. For example:
false || true #=> true
A ! (“not”) reverses the logical state of its operand: if a condition is true, then ! will make it false; if it is false, then ! will make it true. For example:
!true #=> false
!false #=> true
To check if two values are equal, we use the comparison operator represented with == (“double-equal-sign”). If two values are equal, then the statement will return true. If they are not equal, then it will return false. For example:
1 == 1 #=> true
1 == 7 #=> false
Other Comparison Operators
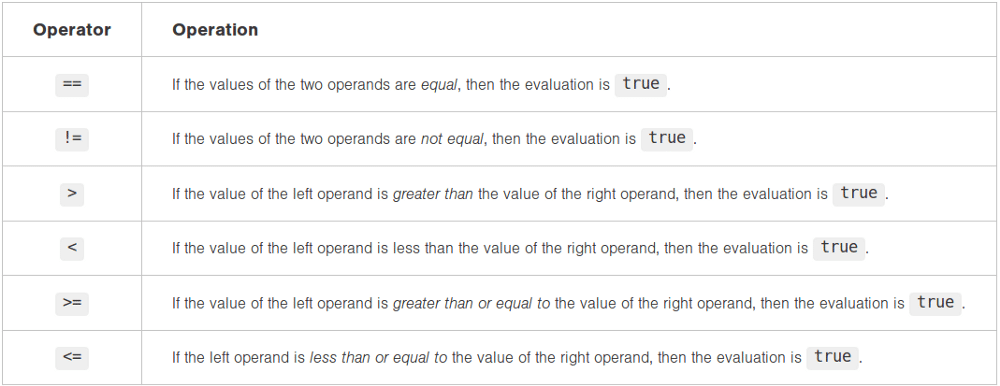
Symbols
Symbol is similar to String but a symbol can’t be changed. It is a thing that has both a number (integer) representation and a string representation. is written like this: :something.
The symbol, however, is immutable. This means that its state can’t be modified after it is created and it will always be the same size in memory. The string referenced the same symbol many times.
First we will see what class is uses a symbol:
:hello.class
=> Symbol
"hello".class
=> String
You can also use the String methods like upcase, downcase and capitalizeon Symbols.
:hello.upcase
=> :HELLO
:HELLO.downcase
=> :hello
:hello.capitalize
=> :Hello
You can’t modify Symbol. You will get NoMethodErrorerror when you try to modify. See below..
:hello << " world"
=> NoMethodError: undefined method '<<' for :hello:Symbol
Symbols are better for performance
When you create two String objects with the same value, those two objects are treated as two different objects. When you create a Symbol, referencing the Symbol will always use the same object. See below results.
"philip".object_id
=> 70288511587360
"philip".object_id
=> 70288504327720
:philip.object_id
=> 539368:philip.object_id
=> 539368
When should you use Symbols?
- To Identify Something: You should use Symbols when you want to identify something. For example a good use case of Symbols is for the keys of a Hash:
person = {:name => "John"}
Or you can defines for String as:
person = {"name" => "Philip"}
You can also used as the name parameters of a method:
Person.age(:John => "31")
Used in method calls
Another use case is when you pass a Symbol into a method call, for example:
people = ["John", "Mike", "David"]
=> ["John", "Mike", "David"]
people.send(:pop)
=> "David"
When you send the Symbol :pop into the method send, the pop method will be called on the object.
Another way, you can use Symbol like
people.respond_to?(:map)
=> true
Numbers
Generally, we use two types of numbers Fixnum and Float. Fixnum known as an Integer. A number with no decimal point, e.g. 52. Float is basically with decimal points. e.g. 34.09988776.
Class Hierarchy
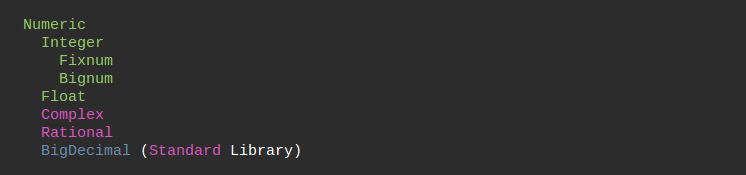
- Ruby has two categories of numbers - integers and floating-point (also called floats).
- Integers are whole numbers that can be positive or negative but cannot be fractions.
- Depending on their size, integers can have the class Fixnum or Bignum.
- Floats are numbers with a decimal place.
Examples:
x = 5.5
x.class
#=> Floatx = 5
x.class
#=> Fixnumx = 11122233344455566677
x.class
#=> Bignum
Strings
In programming language, a string is a text. String is an object that represents a specific text. E.g. your name, paragraphs of this blog, strings are everywhere.
There any many ways to define a String but most common way is double quotes(” “) and single quotes(‘ ‘)
“This is one String”
‘And this is another one.’
Here are some methods we use to define a String in daily basis.
That’s it. These are the basic details and it’s usage of Ruby data types which we are using on a daily basis. I will explain all these data types with its methods in detail in my next blog.
Thanks for reading.
Click here for more RoR blogs…
At BoTree Technologies, we build enterprise applications with our RoR team of 25+ engineers.
We also specialize in RPA, AI, Python, Django, JavaScript and ReactJS.
Consulting is free – let us help you grow!
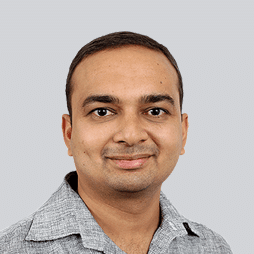