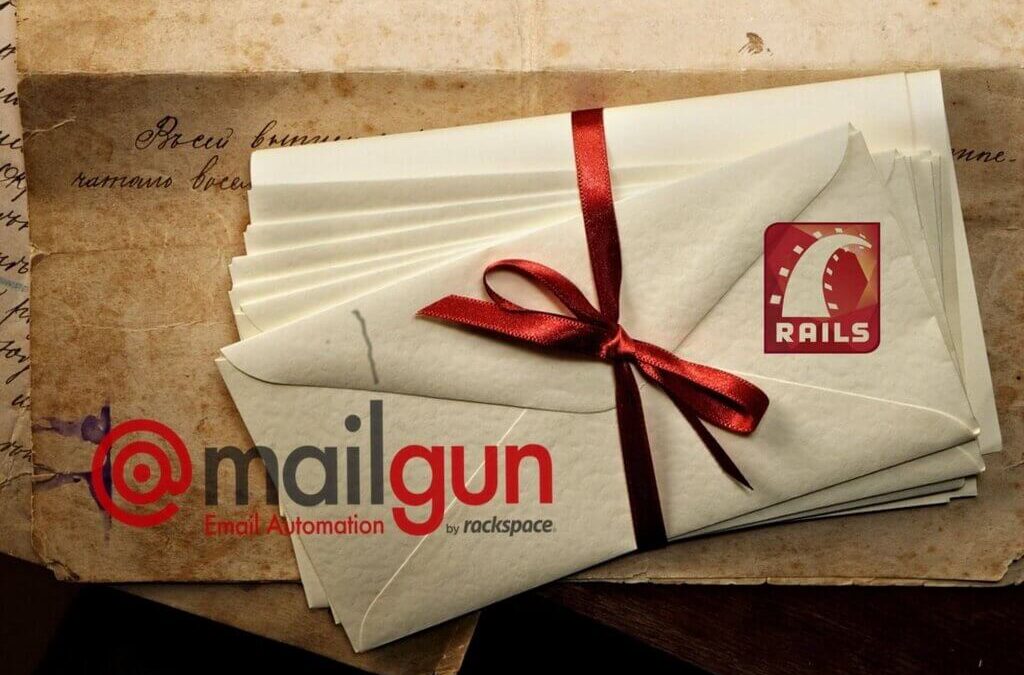
Send Automated Email in Ruby on Rails with Mailgun
Assumptions
You have a Rails project and you want to send an email when a certain event occurs. Lets assume that when user sign up you want to send welcome email to user.
Sign up for Mailgun
Mailgun is a developer tool and API for sending transactional emails. You get 10,000 emails per month for free.
Create an account and add a subdomain to start.
Set up Ruby on Rails Action Mailer
Action Mailer enables you to send emails from your application using mailer classes and views.
Generate your mailer by typing this in Terminal:
rails g mailer model_mailer new_user_notification
Here model_mailer
is the name of the mailer and new_user_notification
is the method.
This create following files.
model_mailer.rb
is where the logic will go for sending email and new_user_notification.text.erb
is the content of the email that will be sent.
Configure Your Development Environment
Log in to Mailgun and click on your domain to find your SMTP settings.
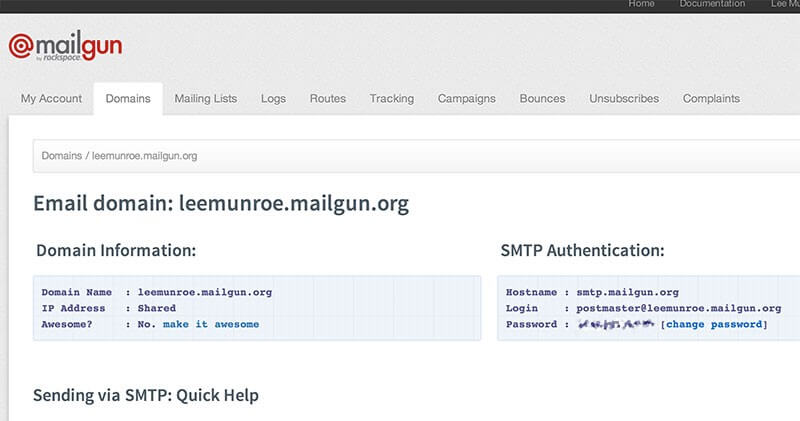
Now open up config/environments/development.rb
Add the following:
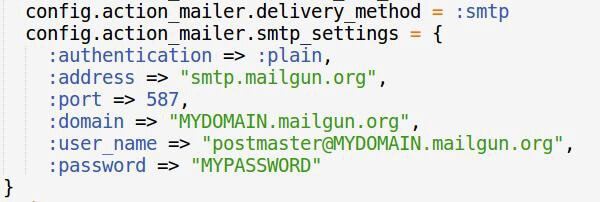
Be sure to replace MYDOMAIN with your Mailgun domain.
Add logic to send the emails
Open up app/mailers/model_mailer.rb and change the default from email:
default from: “me@MYDOMAIN.com”
Then define your new_user_notification method:

Couple of things to note above:
- The “to:” is the email recipient. This could be you or a user, depending on the use case.
- We’re passing the “user” model. This will allow us to use those values in the email content if we wish.
Create a view template (content) for the email
Open up app/views/model_mailer/new_user_notification.text.erb
In this view we add the body content of our email.

Send the email
Last thing to do is to call the email method from your controller.
Whenever you want to send this email, in this case when a user sign up, call this mailer method:
ModelMailer.new_user_notification(@user).deliver
To clarify, your full create action might look something like this:
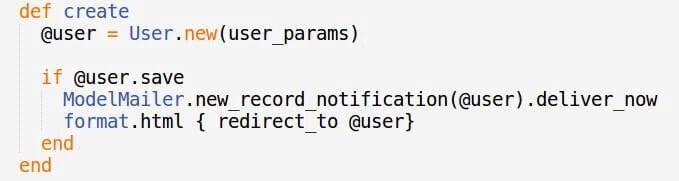
All Done
Now you can use Mailgun’s logs to check sent and delivered emails.
At BoTree Technologies, we build enterprise applications with our RoR team of 25+ engineers.
We also specialize in RPA, AI, Python, Django, JavaScript and ReactJS.
Consulting is free – let us help you grow!
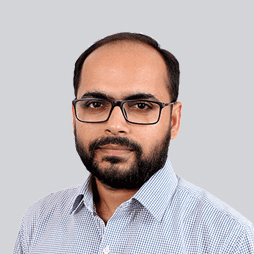