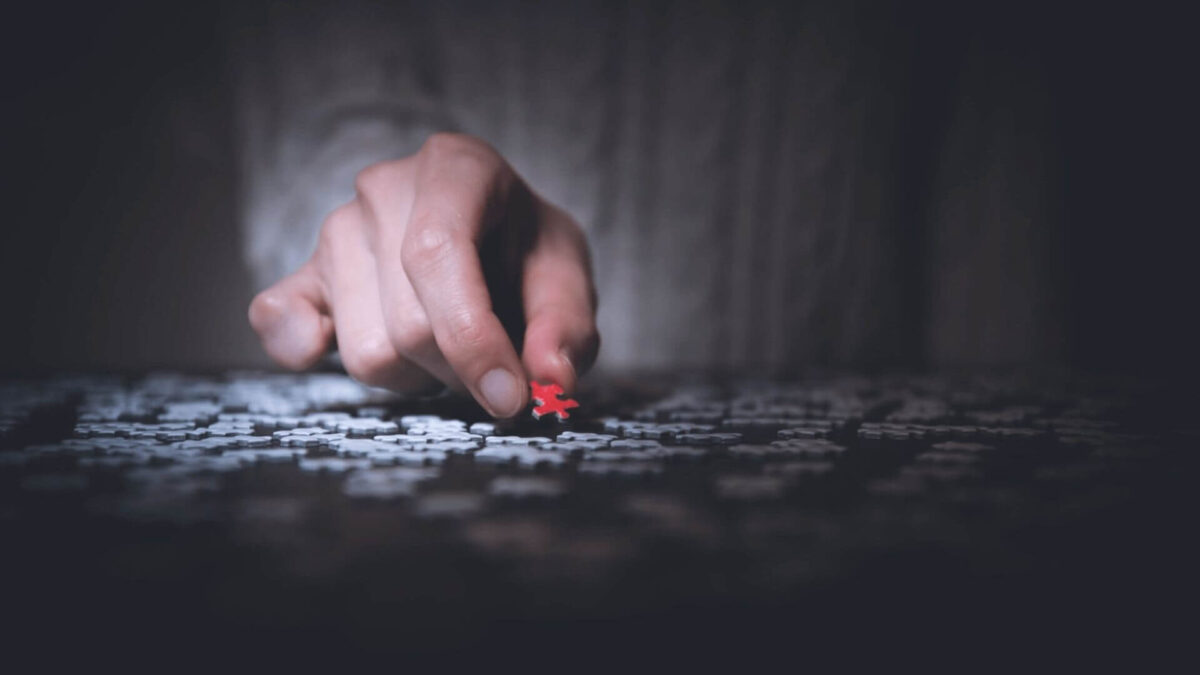
Charge Customers Later Using Stripe With Ruby on Rails
Stripe is one of the most popular payment solutions among business and developers communities. It is equally popular in Ruby community as well.
Stripe Ruby on Rails is useful for payment integrations in online stores and other websites that require payment authorization services.
Rails 6 stripe work together perfectly to ensure that there’s no problem in payment mechanisms. Stripe is becoming a global payment platform, enabling every Ruby on Rails application to take advantage of it.
We are building a health product in Ruby on Rails where we need to store the credit card details and charge the patient after they visit the doctor. I will share a quick demo of how to integrate Stripe, store credit card details on Stripe securely and charge the customer later. Hang tight!
Add the Stripe gem to your application’s Gemfile:
gem 'stripe'
Then, run bundle install to install the gem.
bundle install
First we need the API keys to integrate Rails Stripe gem in our Rails application. For that, we need to create an account in Stripe and generate the secret key and publishable key.
The application makes use of these publishable and secret API keys to interact with Rails Stripe gem securely. An initializer is a good place to set these values, which will be provided when the application is started.
Create config/initializers/stripe.rb add the following:
Now, we can use the above config to call Stripe API for various payment operations.
Checkout Pros and Cons of Ruby on Rails
Let us quickly check the integration working on not using a javascript plugin provided by Stripe. Stripe provides a drop-in the script to add nice looking and secure checkout page in any web application which collects credit card number, CVV and expiry date of the card from the customer
A data-key attribute will be the publishable key that we got from Stripe.
We just need to put this script in a view file where we need to checkout the page. When the view files get rendered, you will have one button for payment like below.
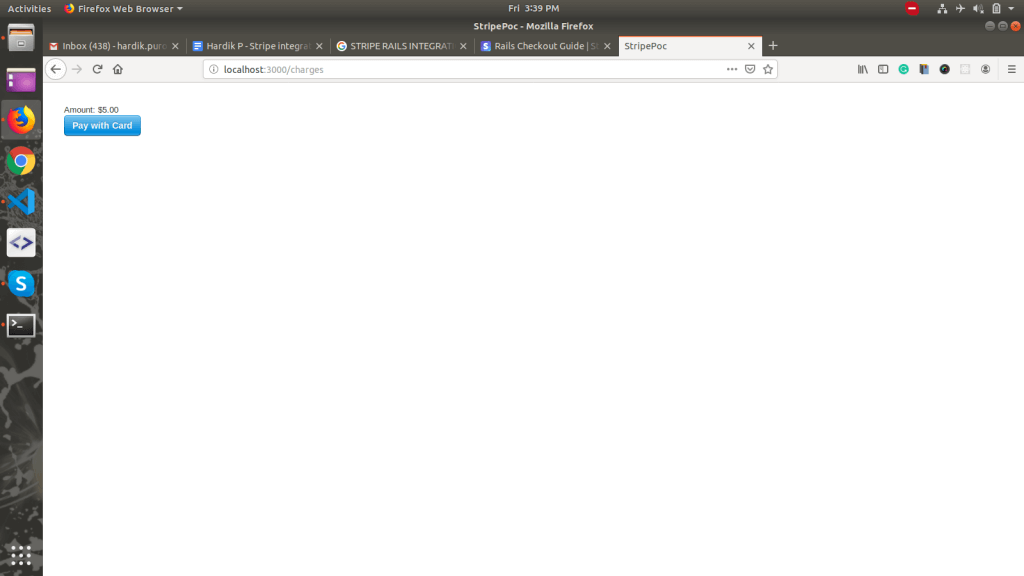
On clicking the button, you will have a popup asking for credit card details like below.
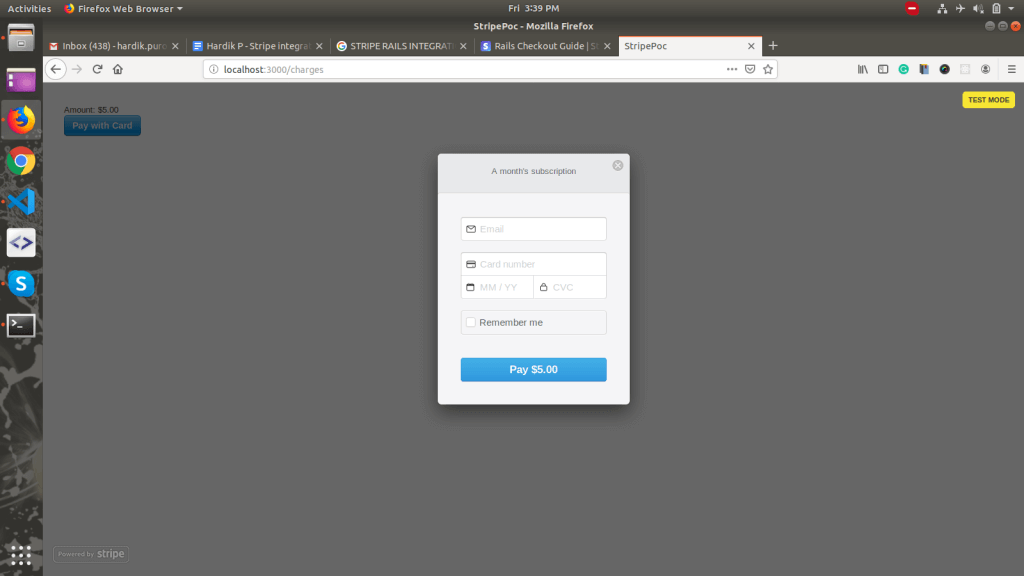
For testing you can use the following details:
Credit Card Number: 4242 4242 4242 4242
CVC: 123
and any future month and year for the expiry date.
Fill in the details and submit. The payment process will be done by the Stripe directly and on the successful operation, it will redirect to the path mentioned in the form above. You should receive request params like below after Striper redirects you back.
Now, we don’t want to ask the user for the credit card details every time he should be charged. Also, storing credit card details on your Rails app is very challenging(PCI Compliance) if it’s not your core feature of the app. Stripe does it very well and follows all compliances required. So let’s leverage that feature to securely save credit card details for customers to charge them later.
We first need a Stripe authentication token for every customer that we want to charge and store it in the database of our app.
To get the authentication token, we first need to generate the Stripe token and then only we will be able to create the customer in Stripe using the token.
Following API call is used to create the Stripe token
Collect the Stripe token from the response
by access it via response[: id]
. We will use this token for creating the customer for the particular user, so we will have the customer key that we will store in the database for processing future payments for that customer.
We will find the customer token using the customer.id
, we need to store this customer.id
in our database.
Now, we have the customer token of the user so let’s proceed to the payment. We will use the charge API as follows:
We will have the response of the API call for about the payment details in charge.
That’s it!
Now we can do as many payments using customer token stored in our database.
Github link: https://github.com/HardikUPurohit/stripe-integration
Read also: Top Reasons Why Businesses Should Invest in Ruby on Rails Development
Happy Coding!!
Consulting is free – let us help you grow!
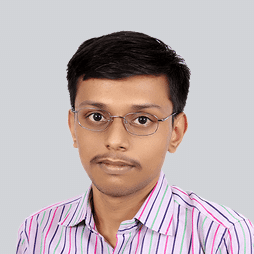