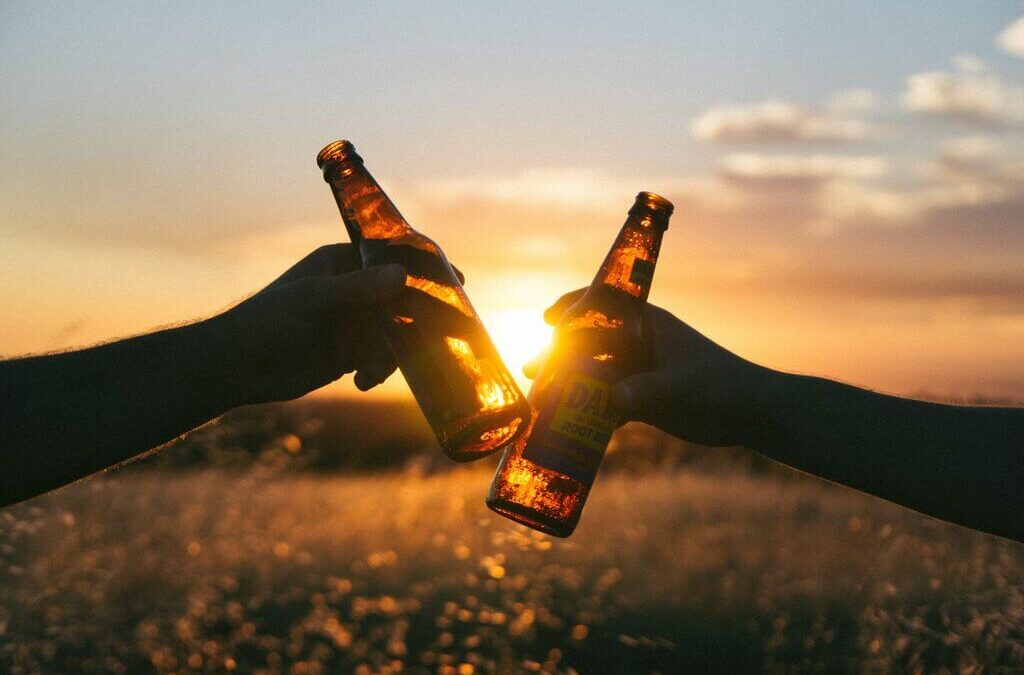
Ruby on Rails: BigCommerce Integration in Simple Steps
BigCommerce offers a simple solution to start an online store. It provides more than 400 features for your online store, and maintenance & configuration of your store is easy and fast.
BigCommerce has its own application store where you can find thousands of useful application/plugins to enhance user experience and workflow (eg. register the order your ERP as it gets created) and access them with a single click. They provide REST APIs and official SDKs for Ruby, PHP, C# and Node.js which makes application development easy. You can easily create and integrate your powerful eCommerce plug-in using their developer friendly platform and reach thousands of merchants.
In this article we are going to create a Ruby on Rails application to manage BigCommerse product management and integrate it with BigCommerce platform. We will create a Rails application to access the list of all products from merchant’s store and perform operations like create new product, update product details and delete product. We will also understand what is webhook, how to register and handle it from the Rails application.
First of all we need to create a trial store on BigCommerce site. Visit BigCommerce and click Get Started button on top right corner.
You will be redirected to Admin page of your store after signing up and there you can manage all the things of your store.
Now your store is ready for use.
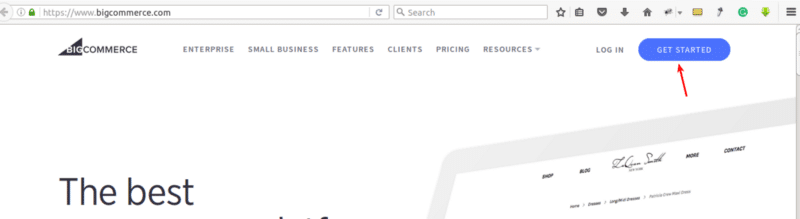
Let’s create an application in developer’s account on BigCommerce. Go to Bigcommerce Developer platform to create one.
Now click My Apps link on top right corner of the home page and login with your credentials of big commerce account you created previously. After successful login attempt, you will be able to see the login page with no application created. Now create your first application here.
I created an application and name it EasyProductManagement.
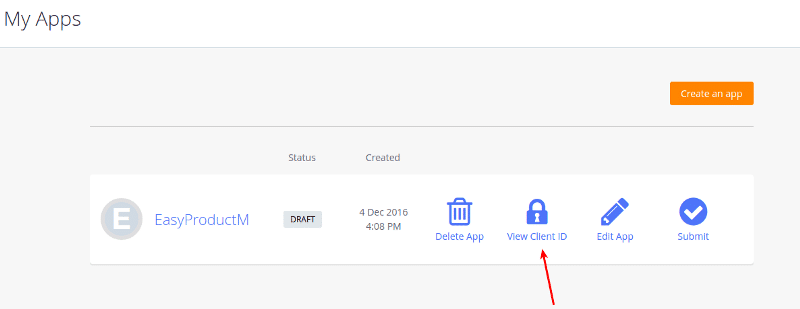
Click on the View client ID icon and you can see a popup containing BigCommerce client ID and BigCommerce client Secret token. We will use it later on in our Rails app.
We have a BigCommerce application with credentials ready for use to access our store using Rest API.
Let’s move to the Rails part now.
First of all, Create a new Rails application and add these two gems in your gemfile.
gem 'omniauth-bigcommerce' gem 'bigcommerce', '~> 1.0.0'
omniauth-bigcommerce – This gem provides a dead simple way to authenticate to Bigcommerce using OmniAuth. It implements OmniAuth Bigcommerce Strategy.
Bigcommerce – This is the official BigCommerce API Ruby client to support their Stores API.
Now run bundle install command
Create a config/initializers/omniauth.rb
initializer:
Now, create one omniauths controller to handle callbacks from Bigcommerce application. Create three routes as given below which is mandatory for your application. We will use this later on while configuring BigCommerce App.
Add these routes to your route file.
get '/auth/:name/callback' => 'omniauths#callback' get '/load' => 'omniauths#load' get '/uninstall' => 'omniauths#uninstall'
Here,
/auth/:name/callback – This URL handles installation process callback. When someone installs your application first time. From here you can get store details and store it into your database.
/load – BigCommerce loads your whole Rails application into one iframe. So whenever any store owner load your application, this method will be called first.
/uninstall – It is important to implement this method to handle data removal when a store owner removes your application.
Add those methods in omniauths controller. I am not going to show implmentation here. You can simply visit my Github repository to see the implementation.
Now create a model Store with fields like, store_hash, access_token, scope, username, email and run migration.
You can load your Rails application in iframe if the hosting server has SSL certificate otherwise you will not be able to integrate your app with BigCommerce. heroku is one option we have but it is very time consuming that you do some change, then deploy it to heroku and then test. So to avoid this hassle, we can use ngrok.
Setup ngrok in your local machine and start it. It will provide you one public URL which tunnels the packets to your localhost so your problem is now resolved!
After setting up ngrok, run below command to start ngrok on your machine.
$ ./ngrok http 3000
Once it starts, you will get an ssl certified public link like give below.
You can access your localhost on it and you can use it to configure your BigCommerce app as well.
So now we have to configure our URLs to our BigCommerce application. We have three URLs here in hand for callback, load and uninstall.
Go to your big commerce developer portal, login and open your newly created application.
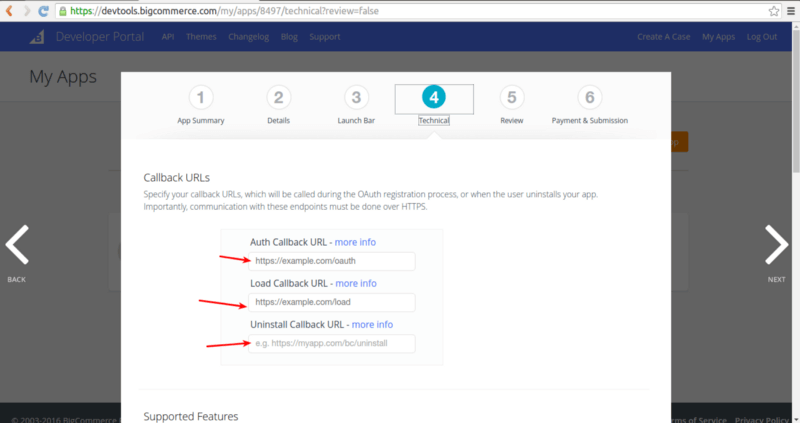
Add your three complete URLs path here.
Now we have to change the scope of the application so that we can access store of the owner with all required permissions.
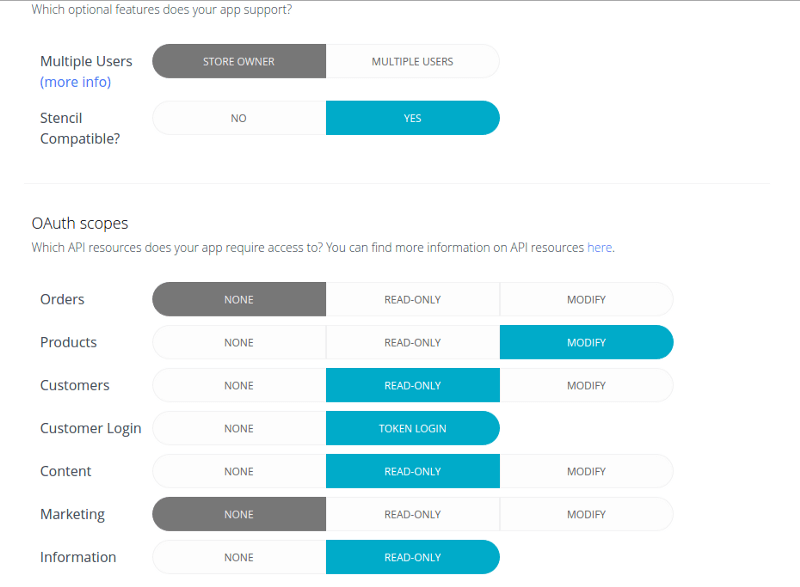
After changing the scopes update the app and close popup.
Now, Go to your Store’s admin panel, go to Apps > My Apps > My Draft Apps > Your App.
You can see your application here in list. Click install button and continue.
It will show a page for asking permission. Just confirm and continue. Once you confirm, it will send callback to callback method of your omniauths controller. From callback method we can store the token to our Database and Store related information. then we render our home page to this BigCommerce iframe. So now you should see your Rails application’s page. Please check the github repository for complete implementation of omniauth controller.
If you can’t see anything on the screen and getting error of load denial due to some headers like given below in image then add one method in after_filter of application controller like given below.
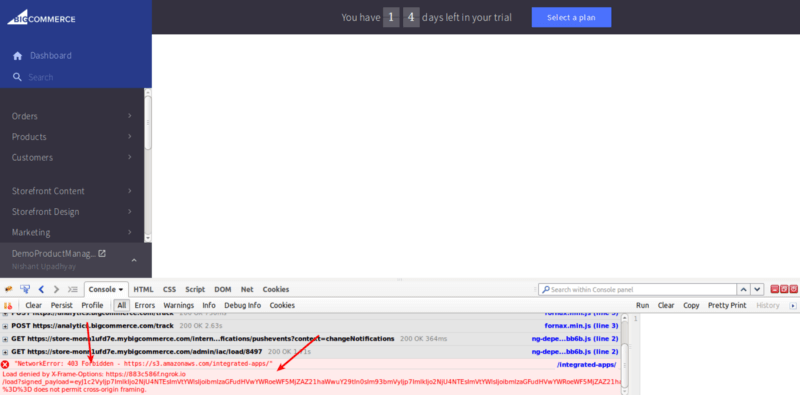
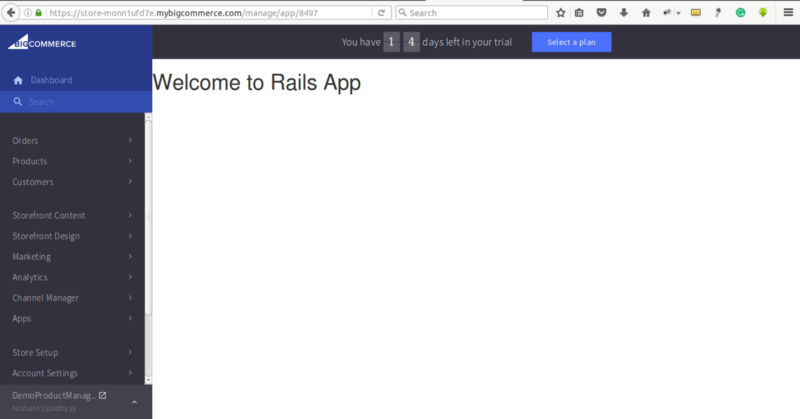
Finally, Integrating Rails application with Bigcommerce is complete.
In next article we will use BigCommerce API to interact with BigCommerce and will also check how to play with webhooks in BigCommerce so keep reading!!!
At BoTree Technologies, we build enterprise applications with our RoR team of 25+ engineers.
We also specialize in Python, RPA, AI, Django, JavaScript and ReactJS.
Consulting is free – let us help you grow!
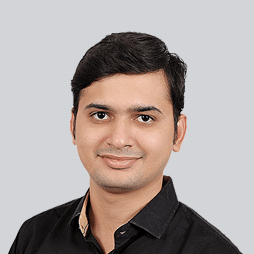