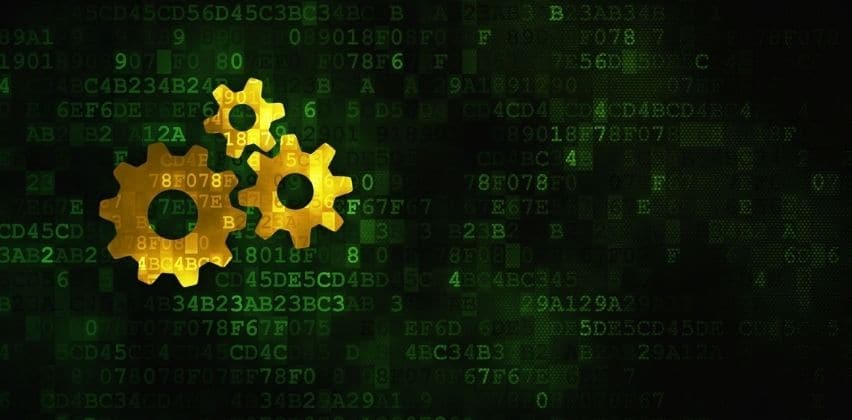
Slugs in Rails using Friendly ID Gem
FriendlyId is a very good gem which allows you to replace ids in your URLs with strings. This lets your Rails app work with ‘friendly’ URLs like ‘myapp /users/john’ as opposed to ‘myapp/users/1’.
Ruby on Rails development company considers this gem as most essential for Rails applications due to its ability to make links memorable.
Let’s start with how to use this gem in a Rails application by following below.
Installation
- The very first step you have to follow is to install the gem.
- Add in your Gem file:
gem 'friendly_id', '~> 5.2' # Note: MUST use 5.0.0 or greater for Rails 4.0+
- Then execute ‘bundle install’.
- Next step is to add a slug column to the model on which you want a friendly_id.
- Here, I have a model called “Group” on which I am going to add a slug column.
rails g migration add_slug_to_groups slug:string:uniq
rails db:migrate
- In
db/migrate/add_slug_to_groups.rb
you have the following:
add_column :groups, :slug, :string
add_index :groups, :slug, unique: true
- Now open the rails console and generate a configuration file for friendly_id.
rails g friendly_id
- If you are implementing friendly_id in your existing model, then you have to run the following command in the rails console to generate their slugs.
Model.find_each(&:save)
- In my case,
Group.find_each(&:save)
- Let’s extend or include the
friendly_id
module in our model to access the methods defined in it. - In models/group.rb, we have a name column for the group on which we are going to add a friendly_id.
class Group < ApplicationRecord
extend FriendlyId
friendly_id :name, use: :slugged
end
- Generally, some people add options like above
use: :slugged
. If you want to configure friendly_id for your all models then you can set default in the initializer. - In config/initializers/friendly_id.rb
FriendlyId.defaults do |config|
config.use :finders
config.use :slugged
end
- Now , you can write in any model friendly_id without using
use: :slugged
.- Create a new group by:
Group.create! name: “tech lead”
- In controller, change the Group.find by below:
@group = Group.friendly.find(params[:id])
- Now you can check your local server by finding a record and check the URL it looks like :
localhost:3000/groups/tech-lead
- Uniqueness feature of friendly_id:
- There is a case when two records have the same slug, at that time which record should you prefer?
- But this problem is solved by friendly_id itself.
- When two records have the same slug then friendly_id appends UUID to the generated slug to ensure the uniqueness of the record.
- i.e.
group1 = Group.create :name => "Tech lead"
group2 = Group.create :name => "Tech lead"
group1.friendly_id #=> "tech-lead"
group2.friendly_id #=>"tech-lead-f9f3789a-daec-4156-af1d-fab81aa16ee5"
- You can also override FriendlyId::Slugged#should_generate_new_friendly_id? To control exactly when to generate new friendly_id.
- i.e.
class Group < ApplicationRecord
extend FriendlyId
friendly_id :name, use: :slugged
def should_generate_new_friendly_id?
name_changed?
end
end
This is how you can add friendly_id to your models to make the user friendly and memorable URLs. and Also you can customize the methods of the FriendlyId module by extending it in your model.rb file.
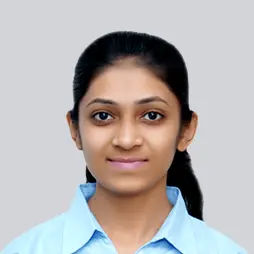