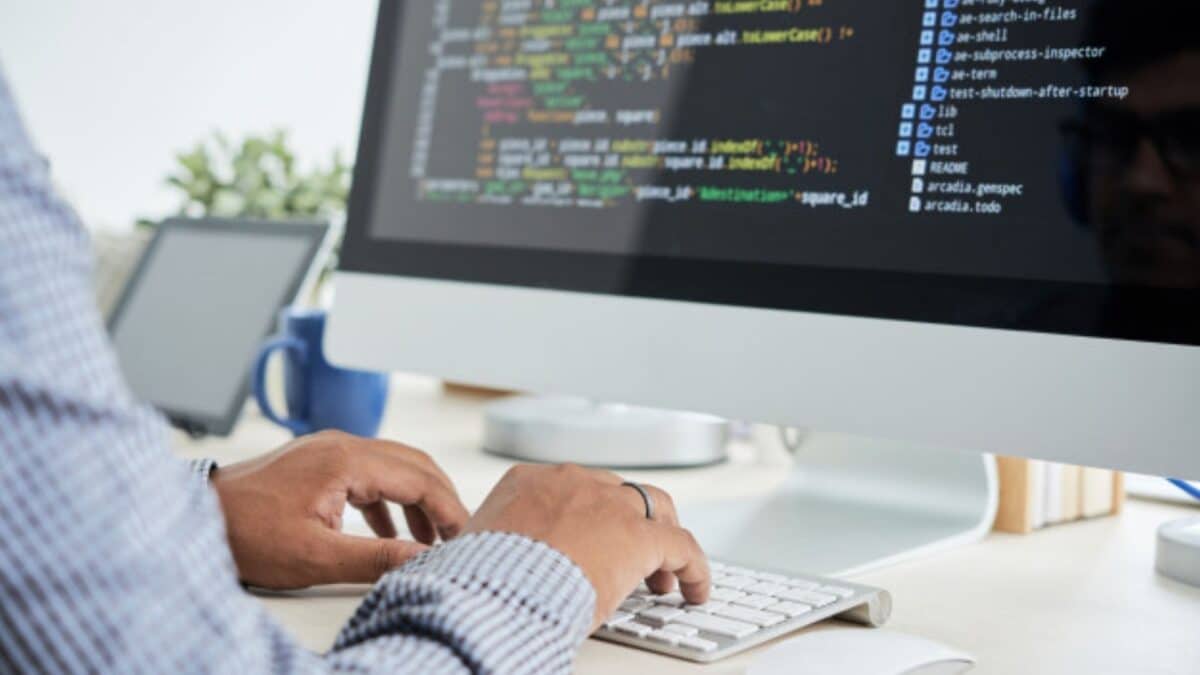
Installation and Usage of Rails MongoDB
Rails MongoDB : Introduction
MongoDB is a document database with the scalability, flexibility and the querying and indexing of the records.
In this blog, we will cover how to install it in Ubuntu 16.04, configure and use it with Rails 6 application.
1. Install MongoDB with Rails in Ubuntu 16.04 LTS
The steps are for Ubuntu 16.04 but you can install in other versions as well.
Follow below steps in your terminal to install it from the source.
sudo apt-key adv --keyserver hkp://keyserver.ubuntu.com:80 --recv EA312927
echo "deb http://repo.mongodb.org/apt/ubuntu xenial/mongodb-org/3.2 multiverse" | sudo tee /etc/apt/sources.list.d/mongodb-org-3.2.list
After adding this repo update the packages
sudo apt-get update
Now, install mongo db using below command
sudo apt-get install -y mongodb-org
If you face any issue like below
WARNING: The following packages cannot be authenticated!
mongodb-org-shell mongodb-org-server mongodb-org-mongos mongodb-org-tools mongodb-org
E:There were unauthenticated packages and -y was used without --allow-unauthenticated
Then install it using the below command. Please note the risk of overriding the authentication warning here.
sudo apt-get --allow-unauthenticated install -y mongodb-org
2. Configuration and Starting the Services
After you are done with the installation, configure and start the service using below commands in your terminal
sudo systemctl start mongod
Check if the service has properly started or not using below command
sudo systemctl status mongod
Using below command you can start it automatically when your system starts
sudo systemctl enable mongod
Now, MongoDB is set up and services are running. Let’s see how to use it with Rails 6
Read Also: Notable ActiveRecord Changes in Rails 6
3. MongoDB Setup with Rails 6
Create a new rails application using below command:
rails new mongorails --skip-active-record --skip-bundle
We have used --skip-active-record
dependencies as we are not using it. We also used --skip-bundle
to skip gem installations as we need to change the gemfile to add MongoDB dependency.
If you are going to to use RSpec for testing then you can create your application using below command by skipping the default testing framework of rails
rails new mongorails --skip-active-record --skip-bundle --skip-test --skip-system-test
Now, go to your project directory and add mongoid gem in Gemfile. Mongoid is the officially supported ODM (Object-Document-Mapper) framework for MongoDB in Ruby.
gem 'mongoid', '~> 7.0.5'
NOTE: Mongoid 7.0.5 or higher is required to use Rails 6.0.
Run below command to install the gem
bundle install
Run below command to generate a default configuration
bin/rails g mongoid:config
This command would create a mongoid.yml
file in config
directory which is a replacement of database.yml
which rails create by default for us.
It is recommended to change server_selection_timeout
from 30 seconds to 1 second for the development environment. You can change it in mongoid.yml
Now, start rails server to check if everything is set up and configured correctly
rails s
If you see below screen after visiting localhost:3000
in your browser then everything is good so far.
4. How to use MongoDB with Rails
Now, let’s create scaffolds using rails and check how the Model is working with MongoDB.
We will create a small application where people can create posts and can comment on it.
Creating Posts
Create a post using scaffolding. Yes, you can use scaffolding with MongoDB as well, and it would create all necessary files as it does for other databases.
rails generate scaffold Post title:string body:text
The important point to note is that we do not have a db folder and there is no need to run migrations.
Post model will look like this
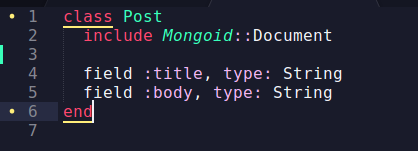
Now, head to the browser and visit localhost:3000/posts
and you will see a list of posts and you can create/update/delete the posts. Same old rails stuff 🙂
Once you create the post it will fire insert query as below:
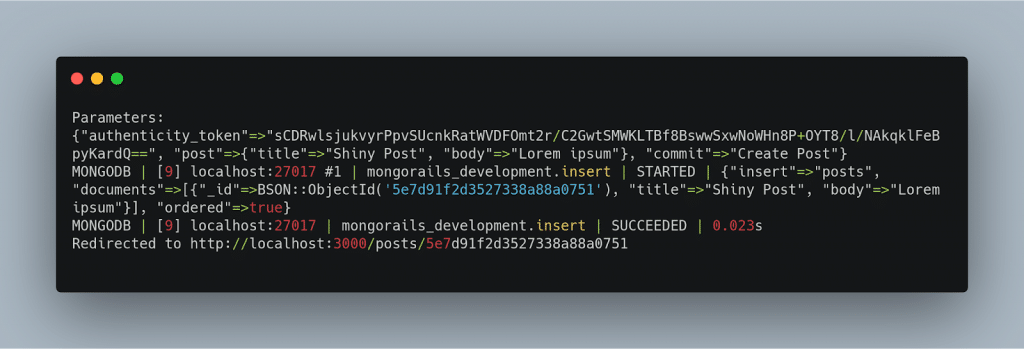
You can notice that it has first found the database name mongorails_development.insert
and then used the below syntax to insert the record to the posts
table.
{"insert"=>"posts", "documents"=>[{"_id"=>BSON::ObjectId('5e7d91f2d3527338a88a0751'), "title"=>"Shiny Post", "body"=>"Lorem ipsum"}], "ordered"=>true}
And when you visit show path of the app, it would fire below query
mongorails_development.find | STARTED | {"find"=>"posts", "filter"=>{"_id"=>BSON::ObjectId('5e7d91f2d3527338a88a0751')}}
You can find the post from your rails console using below command
Post.find('5e7d91f2d3527338a88a0751')
Creating a comment
Let’s create comments on the blogs
Run below command to create comment and associate with posts using scaffold
rails generate scaffold Comment name:string message:string post:belongs_to
By default, it would generate comment model code as below.
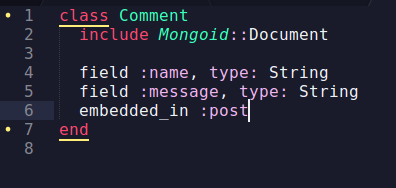
Change embedded_in :post
to belongs_to :post
Open comments/_form
and change the type of field for :message
from text_field to text_area
, as well as the type of field for :post_id
from text_field to hidden_field
Add below code in posts/show.html.erb
to create comments for the post. This will list all the comments for the post and also allow you to add new comments.

Now, create _comment.html.erb partial
in app/views/comments
folder with the code below:
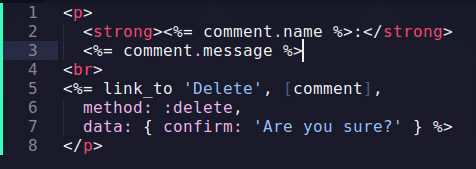
If you want to add a new field to the table just add it using the below line. I have added a new field to posts table using below line
field :slug, type: String
It is that simple!!
You don’t need to declare a foreign key field to comment on the model. It would implicitly create the post_id
to comments table. The naming convention for a foreign key is the same as active records.
That’s it, You have created a small application using MongoDB.
Click here for more details…
At BoTree Technologies, we build enterprise applications with our RoR team of 25+ engineers.
We also specialize in Python, RPA, AI, Django, JavaScript and ReactJS.
Consulting is free – let us help you grow!
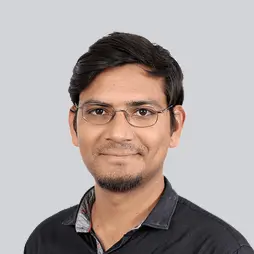