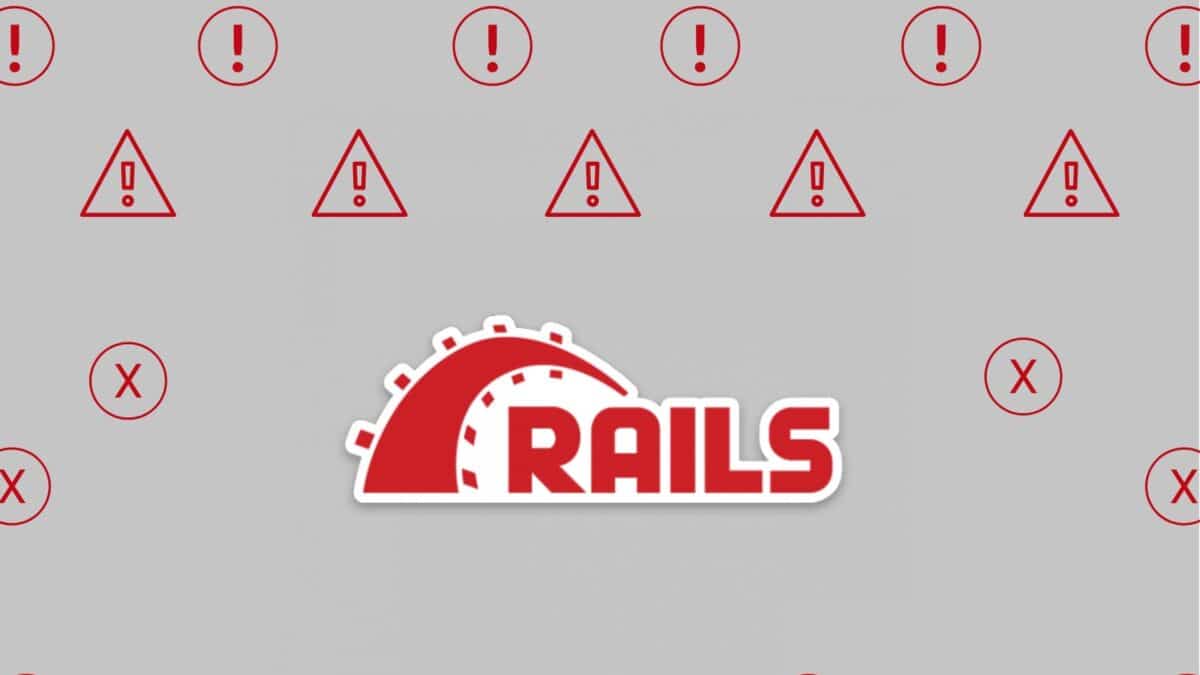
8 Common Ruby on Rails Development Mistakes to avoid
Ruby on Rails is a powerful web development framework that allows building scalable, enterprise-ready applications with complete front-end support. The simplest things about Ruby on Rails development like Convention Over Configuration and MVC pattern make it reliable for highly-functional apps.
Rails developers love the RubyGems that reduce the development time and the need to write excess code. They follow the standard practice and build rapid MVPs, providing companies more time-to-market their products.
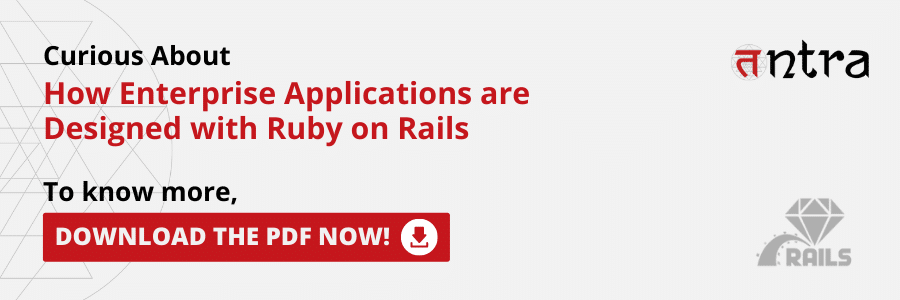
But Ruby on Rails application development is not foolproof. While there may be no inherent mistakes with Ruby on Rails projects, problems generally occur due to human errors. There are certain pitfalls of Ruby on Rails developers that can only be overcome by experience. In this article, we highlight 8 common Ruby on Rails development mistakes that can put your project to a halt.
The 8 most Common Mistakes in Ruby on Rails Development
The following common Rails development mistakes can act as a bottleneck for your project. Don’t worry, we have also provided the solution for these mistakes so that you don’t make them in the future. We provide you the guidance to avoid these mistakes in the Ruby on Rails projects and develop bug-free solutions for your clients.
Mistake #1: Do You Check N + 1 Query Rail
It won’t throw any issue in the application, however, it will affect your application performance.
Eg:
users = User.where(is_active: true)
names = users.map { |user| user.profile.name }
You can avoid N + 1 Query while querying associated records.
Eg:
users = User.where(is_active: true).includes(:profile)
names = users.map { |user| user.profile.name }
Use bullet gem that helps to increase your application’s performance by reducing the number of queries it makes and notifies when to add eager loading.
Mistake #2: Keep Your Configurations Safe
Your application might be using some external services such as Google Calendar, Stripe Payment, AWS and its API keys or credentials are stored in credentials.yml
or secrets.yml
files.
The file often mistakenly gets checked into the source code repository with the rest of your application and, when this happens, anyone with access to the repository can now easily compromise all users of your application.
Mistake #3: Putting too much logic in controllers.
Developers keep too much logic in controllers. Keep the controllers skinny. Do not violate the Single responsibility principle.
What to include?
Session Handling, Model selection, Request parameter management, Rendering/redirecting
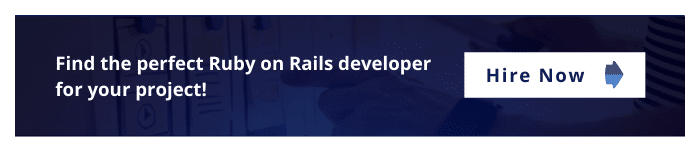
Mistake #4: Putting too much logic in models.
Developers PUT too much logic in models such as email notifications, data conversion from one format to another, etc.
This core functionality can be handled using services like plain ruby objects, so it can be eliminated from ActiveRecord
.
What to include?
configuration (i.e., relations and validations)
Simple mutation methods to encapsulate updating a handful of attributes and saving them in the database.
Access wrappers to hide internal model information.
Eg: full_name that depicts a combination of first_name
and last_name
.
Sophisticated queries, you should never use where
the method or any other query building outside the model class.
Mistake #5: Blocking on calls to external services
3rd party providers of Ruby on Rails applications usually make it very easy to integrate APIs, but sometimes may run slow.
So, to avoid blocking on calls, rather than calling services from your Rails application, you should move some part of code to a background job.
For queuing these API services, you should use Delayed Job, Sidekiq etc.
Mistake #6: Improper Predicate Method Usage
A predicate method is any method that syntactically ends with a question mark (?) should return a true value.
When you are creating a predicate method, it’s important to know its main purpose.
It should be named for what it performs, it should not contradict the name with the action it performs.
For eg, to check whether the user has books available or not, you should define the predicate method in the user model as shown below:
def books_available?
self.books.present?
end
Mistake #7: Not using memoization:
Memoization is a technique used to speed up your accessor during Ruby on Rails development. It caches the results of methods that do time-consuming work or variables initializing.
Uses ||=
operator to initialize the cache variable.
It can be used to initialize the variables from some value in parameters, or the associated records.
Eg:
def google_calendar_event
@event ||= GoogleCalendarEvent.find_by(event_id: params[:event_id])
end
Mistake #8: Follow Proper Naming Convention In Ruby on Rails projects
While developing, keep the following points in your mind:
- Table names should be in a plural format to map your model and table automatically.
- The model name should be in singular.
- Also, make sure you are not using reserved names for your class. For example, you can’t use “Class” but you can use “Klass”.
- Controller name should be plural format and if you want to use multiple words then go with Camelcase.
- Follow the default restful routes to reduce the complexity.
Ruby on Rails is a powerful and feature-rich framework for web application development. While Ruby on Rails application may have some limitations, mistakes arise because developers are not paying attention while deployment and debugging.
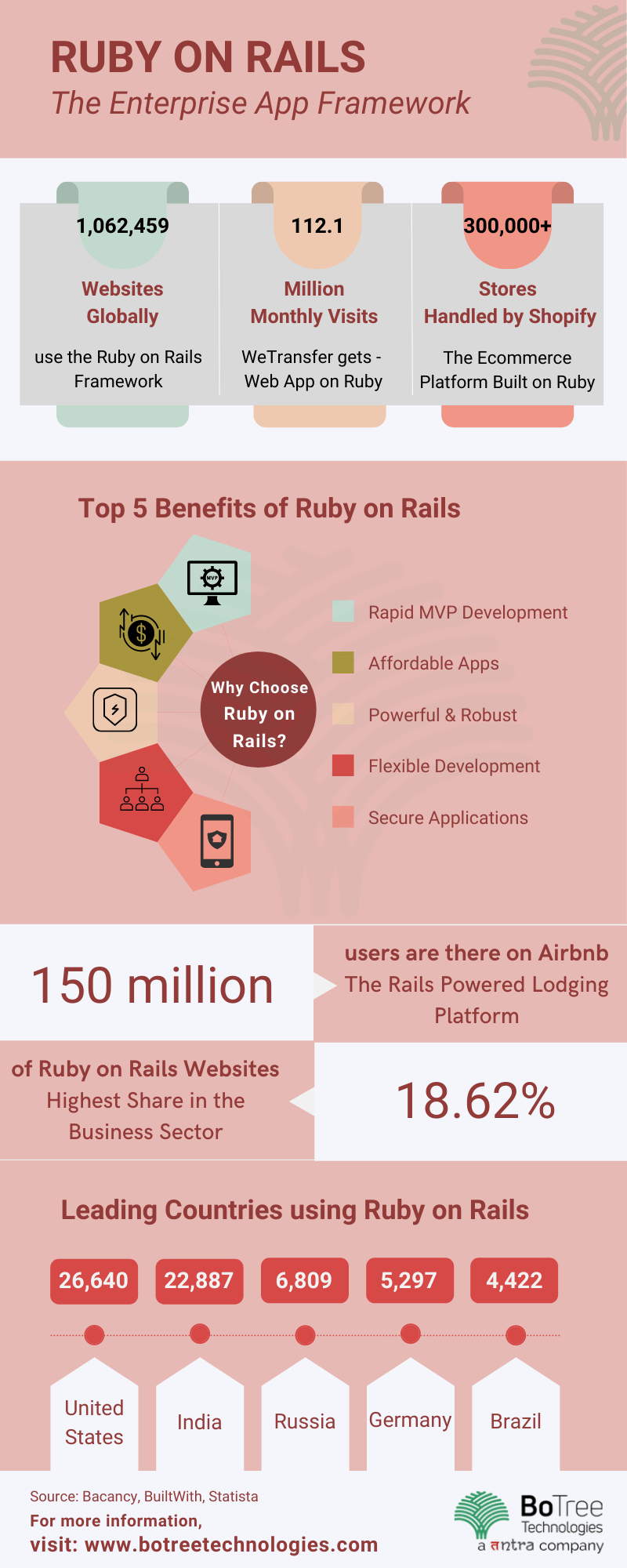
By following the above mistakes, you can build top-notch Ruby on Rails applications. At BoTree, a leading Ruby on Rails development company, we follow the best Ruby practices to eliminate bugs from your application.
Our developers are experienced and have already learned from these mistakes to avoid halting the project. You can hire Ruby on Rails developers from us for rapid & error-free development.
Consulting is free – let us help you grow!
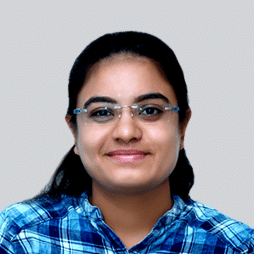